PHP与数据库交互
1.连接mysql数据库
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30
| <?php $link = mysqli_connect('localhost','root','root','xhh'); if ($link){ echo '连接成功'; } echo "<pre>"; $sql = "insert into tb_users values(3,'小灰灰','123456','2020-1-28 17:52:23','云南')"; mysqli_query($link,$sql);
$mysqli = new mysqli('localhost','root','root','database9'); if ($mysqli->connect_errno){ die('连接失败'.$mysqli->connect_errno); }else{ echo '连接成功'; } echo '<pre>'; $sql = "insert into books values(4,'零基础学PHP','PHP','68.80','2017-02-17')"; if ($mysqli->query($sql)){ echo '添加表数据成功'; }
?>
|
2.常用的Mysql语句
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
| create database name; 创建数据库
use databasename; 选择数据库
drop database name 直接删除数据库,不提醒
show tables; 显示表
describe tablename; 表的详细描述
select 中加上distinct去除重复字段
mysqladmin drop databasename 删除数据库前,有提示。
显示当前mysql版本和当前日期
select version(),current_date;
一些常用的mysql语句:比如对数据库的增删改查 增:insert into 表名(字段名) values (字段值) 删:delete from 表名 where 条件 改:update 表名 set 字段名 = 字段值 查:select 字段名 from 表名 where 条件
|
3.将结果集返回到数组
在使用mysql_query()函数执行mysql语句,如果成功的话函数将返回一个结果集,而现在我们要将结果集返回到数组中。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26
| <?php
$link = mysqli_connect('localhost','root','root','database9'); if ($link){ echo '连接成功'; } $sql = 'select * from books'; $result = mysqli_query($link,$sql); while ($rows = mysqli_fetch_array($result,MYSQLI_ASSOC)){ echo '<pre>'; print_r($rows); } ?>
|
4.从结果集获取一行作为对象
除了用mysqli_fetch_array()函数获取结果集中的数据,还可用mysqli_fetch_object()函数实现。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
| <?php
$link = new mysqli('localhost','root','root','database9'); if($link->connect_errno){ die ('连接失败'); }else{ echo '连接成功'; } echo '<pre>'; $sql = 'select * from books'; $result = mysqli_query($link,$sql); $rows = mysqli_fetch_object($result); echo $rows->id; echo '<pre>'; echo $rows->name; ?>
|
5.查询结果集中的记录数
使用mysqli_num_rows()函数,可以获取由select语句查询到的结果集中行的目数。
一般可用于统计一个网站的数据条数目,便于与用户的交互
1
| 语法:mysqli_num_rows($result) $result为查询语句后结果集的变量
|
6.释放内存与关闭连接
mysqli_free_result()函数用于释放内存,数据库操作完成以后,需要关闭结果集,以释放系统资源。
mysqli_close()函数用来断开与mysql服务器的连接。
1 2 3 4
| 一般可以在引入html前端模板后,可以写这俩句 include_once('list.html'); mysqli_free_result($result); mysqli_close($link);
|
7.php避免SQL注入攻击
一般php文件用于连接数据库,发送查询以及检查结果,当使用insert将数据插入数据库的时候,为避免sql注入攻击,需要使用prepare语句进行预处理,然后使用bind_param()函数进行参数绑定,最后使用execute()函数来执行sql语句。
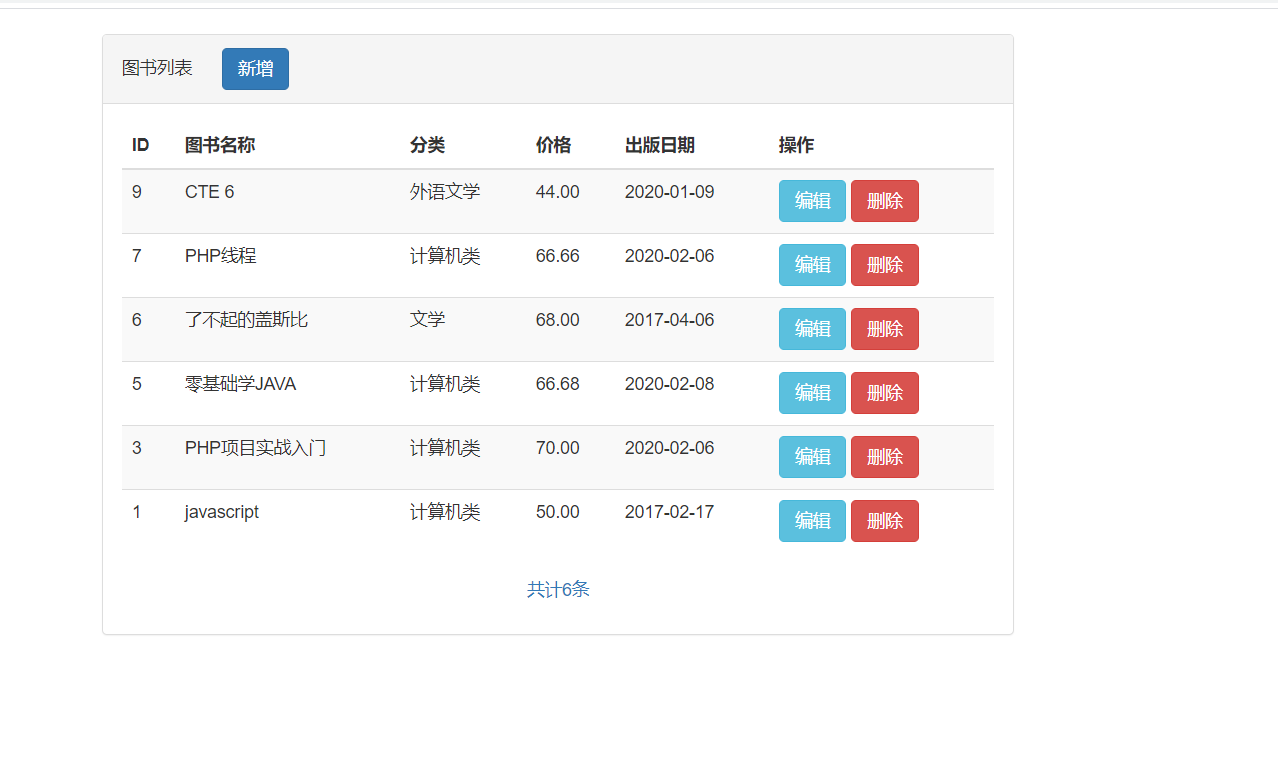
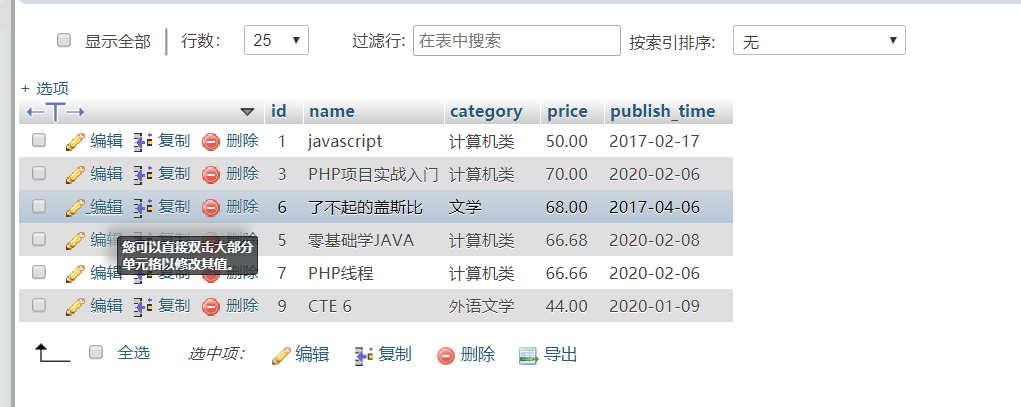
此图为我的database9数据库的books表,我将对它进行一系列插入操作:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28
| <?php $link = mysqli_connect('localhost','root','root','database9'); if ($link){ echo '连接成功'; }
$name = $_POST['name']; $category = $_POST['category']; $price = $_POST['price']; $publish_time = $_POST['publish_time']; $sql = "insert into books(name,category,price,publish_time)values (?,?,?,?)"; $stmt = mysqli_prepare($link,$sql); mysqli_stmt_bind_param($stmt, 'ssds',$name,$category,$price,$publish_time); mysqli_stmt_execute($stmt); if(mysqli_stmt_affected_rows($stmt)){ echo "<script>alert('添加成功');window.location.href='123.php';</script>"; }else{ echo "<script>alert('添加失败');</script>"; }
?>
|